Calculating and Optimizing Transaction Costs in Solidity
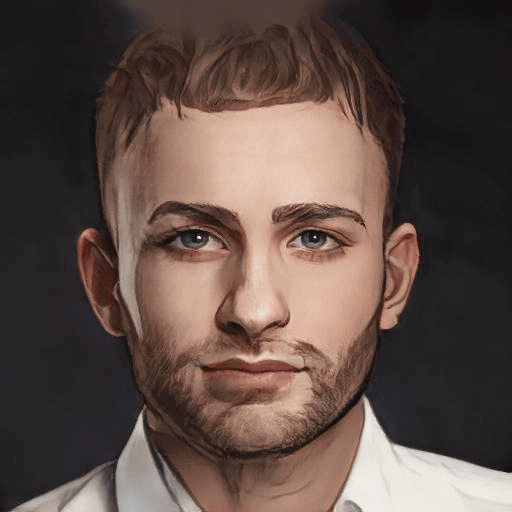
- Published on
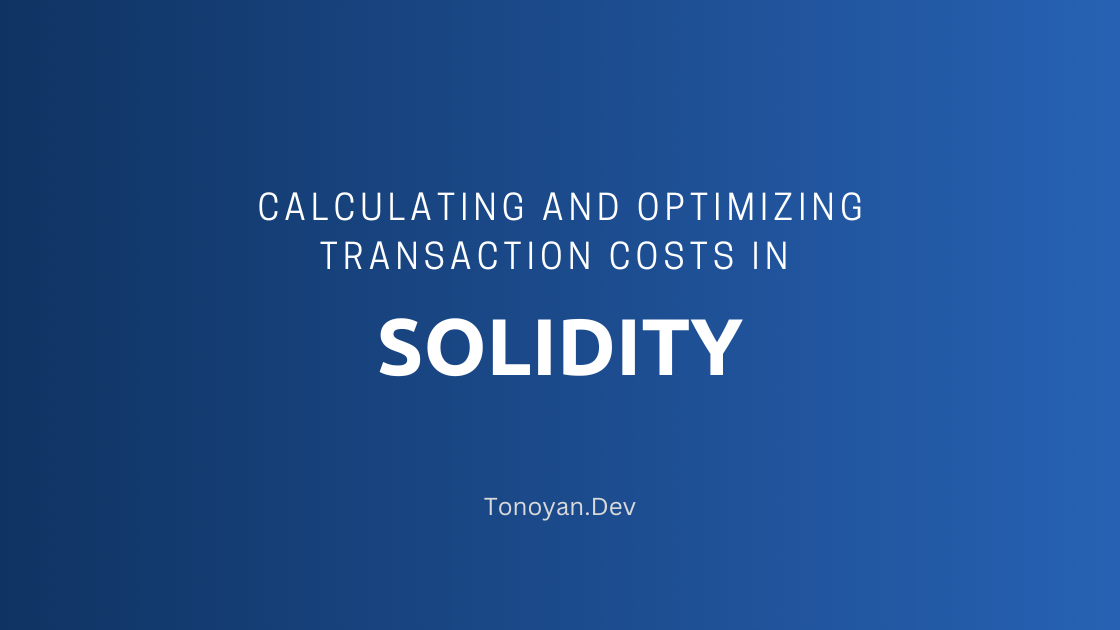
Calculating and Optimizing Transaction Costs in Solidity
In the realm of Ethereum, transaction costs are a central concern. They dictate the feasibility and the economic sense of deploying and interacting with smart contracts. As Solidity developers, we need to understand how to calculate these costs and optimize them to ensure that our dApps are not only functional but also economically efficient.
In this article, we'll delve into popular and less common ways to calculate and optimize transaction costs in Solidity, including the use of assembly and various optimization techniques.
Understanding Transaction Costs in Ethereum
Before we delve into the calculation and optimization of transaction costs, it's essential to understand what makes up these costs in Ethereum. Transaction costs in Ethereum are defined in terms of gas. Each operation in the Ethereum Virtual Machine (EVM) costs a certain amount of gas, and this gas is paid for in Ether.
The total transaction cost is therefore determined by two factors:
1 - Gas Used: This is the total gas that is used by a transaction. It's the sum of the gas cost of all the EVM operations that are executed in the transaction.
2 - Gas Price: This is the amount of Ether that you are willing to pay for each unit of gas. It's typically denoted in Gwei, where 1 Gwei = 10^-9 Ether.
The total transaction cost in Ether is thus calculated as Gas Used * Gas Price
.
Calculating Transaction Costs in Solidity
Calculating transaction costs in Solidity primarily involves determining the gas used by a transaction. There are two main ways to do this:
1 - Estimation Using estimateGas
: The estimateGas
function provided by web3 libraries allows you to estimate the gas used by a transaction without actually sending it. Here's a sample usage in JavaScript:
const gasEstimate = await contract.methods.myMethod().estimateGas({ from: userAddress });
This method provides an estimate of the gas used, but it may not be entirely accurate because the actual gas used may depend on the state of the contract at the time the transaction is mined.
2 - Measurement Using gasleft
: The gasleft
function in Solidity returns the amount of gas still left. You can use this function at the start and end of a function to measure the gas used by the function. Here's a simple example:
function myFunction() public {
uint256 startGas = gasleft();
// Code to be measured
uint256 endGas = gasleft();
uint256 gasUsed = startGas - endGas;
}
This method provides an accurate measurement of the gas used by a function, but it can only be used in tests because it involves modifying the contract code.
optimizing Transaction Costs in Solidity
optimizing transaction costs in Solidity mainly involves reducing the gas used by your transactions. Here are some common and less common techniques:
1 - Use Smaller Data Types: The smaller the data type, the less gas it costs to store and manipulate. For example, using uint8
instead of uint256
where possible can save gas.
2 - Avoid Dynamic Arrays in Memory: Dynamic arrays in memory are much more expensive than fixed-size arrays. If the size of your array does not change, use a fixed-size array instead.
3 - Use External Instead of Public: The external
function type is cheaper than public
because the former reads directly from calldata
while the latter makes a copy in memory.
4 - Assembly optimizations: Assembly allows for lower-level control of the EVM and can be used for optimizations that are not possible
in Solidity. However, it should be used sparingly because it makes the code harder to read and more prone to errors. Here's an example of an assembly optimization:
function assemblyAdd(uint256 a, uint256 b) public pure returns (uint256 result) {
assembly {
result := add(a, b)
}
}
This function uses assembly to add two numbers, which is cheaper than the corresponding Solidity code because it avoids the overflow check.
5 - optimizer Settings: The Solidity compiler comes with an optimizer that can reduce the gas cost of your contracts. You can enable it and configure its settings in your solc
command or your Truffle configuration. For example:
// Truffle configuration
module.exports = {
// ...
compilers: {
solc: {
version: "^0.8.0",
settings: {
optimizer: {
enabled: true,
runs: 200
}
}
}
}
};
The runs
setting is the estimated maximum number of times a contract function will be called. A higher value optimizes more for runtime costs, while a lower value optimizes more for deployment costs.
Conclusion
Calculating and optimizing transaction costs is an integral part of Solidity development. By understanding the cost structure and applying the right optimization techniques, you can ensure that your dApps are economically efficient and more appealing to users.
Remember, while optimization is important, it should not come at the expense of readability and security of your code. Always strike a balance between efficiency, readability, and security when writing smart contracts in Solidity.